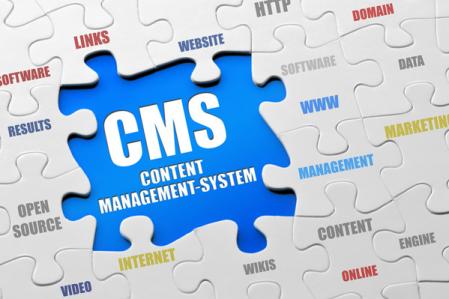
There are a lot of people, who want to write their own site, avoiding use a CMS like WP or Joomla, either because they don't want to update it once in a while, or because they want to learn a programming language.
It is very easy to find a free theme and use it to build your site. In this article, we will see how you can build a simple CMS with Search Engine Optimization (SEO).
Sure, it's easier to use Wordpress or Joomla, but sometimes these systems are very "heavy" for a cheap web hosting and they are having some extra stuff some users don't want. Most people want to just write their articles, thus they are choosing blogger or the free host of wordpress.com to do their work.
But if you want to start learning PHP, you can make your own CMS, which is of course lighter than big CMSs and in many cases, more secure. And of course, it will be your own code and you can sell it to anyone.
Seo Links
Search Engine Optimization (SEO) links are the links who are friendly not only to search engines, but to people too. Is far more easier to remember "site.com/my-page/" from "site.com/index.php?type=blog&id=2". So, in this script, we will add SEO support to our project.
Before starting
Before starting, we have to tell our server to send every request to our index page. To do that, you have to add an ".htaccess" file with the proper commands and of course, your server must support these files.
Open an .htaccess by using any text editor (notepad++ is a good choise) and put the commands from below:
RewriteEngine on
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule ^ index.php [L]
So now, we are sending every request to our index.php file, unless it's a folder or another file already in the server. In this case, the server will return this file instead.
Database
Before continue, we have to create our database tables, so there we can put our data. If you don't know how to create a database, watch this video first.
CREATE TABLE post_categories (
cid smallint unsigned NOT NULL auto_increment,
name varchar(120) NOT NULL default '',
seo varchar(60) NOT NULL default '',
description text NOT NULL,
active tinyint(1) NOT NULL default '1',
posts int unsigned NOT NULL default '0'
PRIMARY KEY (cid)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
CREATE TABLE posts (
pid int unsigned NOT NULL auto_increment,
cid int unsigned NOT NULL default '0',
subject varchar(120) NOT NULL default '',
seo varchar(60) NOT NULL default '',
username varchar(80) NOT NULL default '',
dateline int unsigned NOT NULL default '0',
post text NOT NULL,
draft tinyint(1) NOT NULL default '0',
KEY pid (pid),
KEY draft (draft),
KEY dateline (dateline)
PRIMARY KEY (pid)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
INSERT INTO post_categories (cid, name, seo, description) VALUES (1, 'My Category', 'my-category', 'Test Category');
INSERT INTO posts (pid, cid, subject, seo, post, username, dateline) VALUES (1, 1, 'My Post', 'my-post', 'Test Post
rnThis is a test post.', 'User', UNIX_TIMESTAMP());
The index file
As long as we are sending every request to the index.php file, we have to make this file able to connect to the database and search if there is the data we are looking for. To do this safely, we can add this to our file:
query( "SET NAMES 'UTF8'" );
$query = $db_connection->prepare(
"SELECT p.subject, p.pid, p.post, p.username, p.dateline, c.name, c.seo as c_seo
FROM posts as p
INNER JOIN post_categories as c ON c.cid = p.pid
WHERE p.seo = ?"
);
$query->bind_param( "s", $link);
$query->execute();
$query->bind_result($subject,$pid,$post,$username,$dateline,$name,$c_seo);
$query->fetch();
$query->close();
if($pid == 0)
{
header('HTTP/1.0 404 Not Found', true, 404);
include ('my-404-page.php');
exit;
}
$db_connection->close();
?>
With the above code, we took the element needed from the URL, remove the "/" and used the "urldecode" to decode this element before searching the database.
By using the function "bind_result", we attached the variables to the database fields. Now, we can use these fields to show the data on our page.
';
echo 'Username: ' . $username . '
';
echo 'Title: ' . $subject . '
';
echo 'Post: ' . $post . '
';
echo 'Date: ' . date('d-m-Y', $dateline) . '
';
?>
Of course you can do more things in order to have a fully functional project, like an admin page where we can add, remove or edit our pages for example.
This post is very simple and limited, so you can understand the basics. If this is too much for you, but you don't want to use a heavy CMS, you can choose a flat file CMS for your project.